Project 1f - Moving Obstacles, Collision Detection, Collision Reaction, Report Timing
Goal: The goal of phase p1f is to design obstacles that the human runner can run into.
When the human car collides with the obstacle, this will be detected and simulated as a 2D elastic
collision. Also the race will be timed and the player will be informed of his score
and whether or not he outran the other player.
System: My project was written on Windows XP using Processing (http://processing.org). The Java wrapper used by Processing should make it cross-platform compatible.
Moving Obstacles: For simplicity I decided to make the obstacle be the second player. This creates a challenging game because if the human car collides with the computer controlled car, it will disable the human car momentarily. This allows the computer to advance toward the finish line. The human car has the option of passing the computer (risky) or attempting to go off-road, which will slow down the car significantly. I found this combination to work well.
Collision Detection: Collision detection is done using bounding spheres. An invisible sphere is placed at the origin of each car, and at each step the program determines whether the spheres collide. If they do it initiates the reaction. To detect whether the two spheres collide, I use the principle outlines in this diagram:
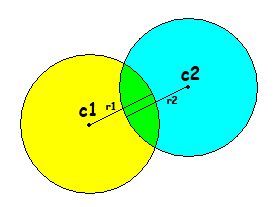
Collisions are detected using bounding spheres.
First the distance is taken from C1 to C1. If this distance is less than R1 + R2, then the spheres must collide. Since taking the distance involves an expensive square root procedure, I square everything which yields the same result at a lower performance cost.
Collision Reaction: For the collision reaction, I decided to make the computer controlled car's weight "infinite" so that it will have no reaction when the human collides with it. Since the human will be disabled for a few seconds, this creates a challenge for the human driver to catch up to the computer. I broke the human reaction into two scenarios. The first scenario occurs when the human car is coming from behind and hits the back of the computer. When this happens, the human's previous position is used to determine the final resting place. Then an animation mode is entered where the human cannot control the car. This animation mode slides the car from the collision point to the final resting place. The car then stalls for a few seconds to make the race more challenging. The second scenario occurs when the computer runs into the human car, sending it bouncing forward. The final human car position is calculated using the next computer car position, and the animation proceeds as described above. I used a linear animation for collisions, but realistically the car would be slowing down. This would be one thing I would improve upon given more time to work on the project.
Report Timing: In order to time the race, I use the framerate command in processing to keep the framerate constant. Since I know the framerate in frames per second, at each frame I can determine how many seconds have elapsed since the start of the race. Unfortunately due to a bug in processing the framerate command seems to be more of a recommendation, which sometimes causes the timer to count faster than usual. I could probably use java time calls to remedy this, but since winner determination works fine I didn't see this as much of an issue. As for determining the winner, at each step I check if a car collides with the finish line (using the same collision detection technique described above) and declare that car the winner. To prevent this from happening at the very beginning of the race, I impose a minimum time in which the cars must be away from the finish line.
Improvements: There are several improvements which I could think of that would make the game more realistic. First of all, it would be nice to have "checkpoints" so that each human car must loosely follow the road, instead of going off-road to skip the track. I would also widen the road to make it "actual size" and create more obstacle cars traveling in the correct direction in each lane. One advanced improvement I never got the chance to implement was a "swaying" camera dolly. This dolly would follow the car more loosely, so that if the car turned sharply it would take a few moments for the camera to catch up. Finally, for control I would implement some kind of gamepad or steering wheel instead of the mouse to make driving feel more realistic.
Applet Instructions:
Drag the vertices to edit the track.
Drag midpoints (small dots) to create a new vertex.
Press 1 to enable editing mode.
Press 2 to enable 2D racing mode.
Press 3 to enable 3D racing mode.
Press UP ARROW or DOWN ARROW in racing modes to change the camera angle.
Press LEFT ARROW or RIGHT ARROW in racing modes to change the camera distance.
Press + or - in editing mode to create less or more interpolated curves.
Use your mouse to control the car. Up/Down = Acceleration. Left/Right = Turn.
Applet:
Source Code: p1f.pde
Demo Video: p1f-demo-divx.avi (5.8 MB, DivX Required)
Demo Pictures:
(click to enlarge)
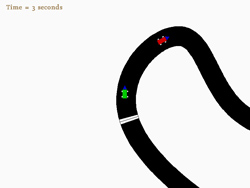
The timer starts when the race begins.
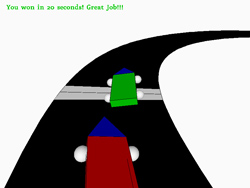
The green human controlled car crosses the finish line first.
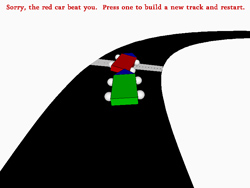
The red computer controlled car crosses the finish line first.
System: My project was written on Windows XP using Processing (http://processing.org). The Java wrapper used by Processing should make it cross-platform compatible.
Moving Obstacles: For simplicity I decided to make the obstacle be the second player. This creates a challenging game because if the human car collides with the computer controlled car, it will disable the human car momentarily. This allows the computer to advance toward the finish line. The human car has the option of passing the computer (risky) or attempting to go off-road, which will slow down the car significantly. I found this combination to work well.
Collision Detection: Collision detection is done using bounding spheres. An invisible sphere is placed at the origin of each car, and at each step the program determines whether the spheres collide. If they do it initiates the reaction. To detect whether the two spheres collide, I use the principle outlines in this diagram:
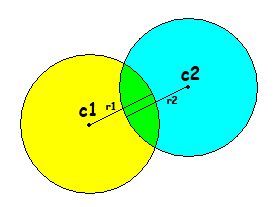
Collisions are detected using bounding spheres.
First the distance is taken from C1 to C1. If this distance is less than R1 + R2, then the spheres must collide. Since taking the distance involves an expensive square root procedure, I square everything which yields the same result at a lower performance cost.
Collision Reaction: For the collision reaction, I decided to make the computer controlled car's weight "infinite" so that it will have no reaction when the human collides with it. Since the human will be disabled for a few seconds, this creates a challenge for the human driver to catch up to the computer. I broke the human reaction into two scenarios. The first scenario occurs when the human car is coming from behind and hits the back of the computer. When this happens, the human's previous position is used to determine the final resting place. Then an animation mode is entered where the human cannot control the car. This animation mode slides the car from the collision point to the final resting place. The car then stalls for a few seconds to make the race more challenging. The second scenario occurs when the computer runs into the human car, sending it bouncing forward. The final human car position is calculated using the next computer car position, and the animation proceeds as described above. I used a linear animation for collisions, but realistically the car would be slowing down. This would be one thing I would improve upon given more time to work on the project.
Report Timing: In order to time the race, I use the framerate command in processing to keep the framerate constant. Since I know the framerate in frames per second, at each frame I can determine how many seconds have elapsed since the start of the race. Unfortunately due to a bug in processing the framerate command seems to be more of a recommendation, which sometimes causes the timer to count faster than usual. I could probably use java time calls to remedy this, but since winner determination works fine I didn't see this as much of an issue. As for determining the winner, at each step I check if a car collides with the finish line (using the same collision detection technique described above) and declare that car the winner. To prevent this from happening at the very beginning of the race, I impose a minimum time in which the cars must be away from the finish line.
Improvements: There are several improvements which I could think of that would make the game more realistic. First of all, it would be nice to have "checkpoints" so that each human car must loosely follow the road, instead of going off-road to skip the track. I would also widen the road to make it "actual size" and create more obstacle cars traveling in the correct direction in each lane. One advanced improvement I never got the chance to implement was a "swaying" camera dolly. This dolly would follow the car more loosely, so that if the car turned sharply it would take a few moments for the camera to catch up. Finally, for control I would implement some kind of gamepad or steering wheel instead of the mouse to make driving feel more realistic.
Applet Instructions:
Drag the vertices to edit the track.
Drag midpoints (small dots) to create a new vertex.
Press 1 to enable editing mode.
Press 2 to enable 2D racing mode.
Press 3 to enable 3D racing mode.
Press UP ARROW or DOWN ARROW in racing modes to change the camera angle.
Press LEFT ARROW or RIGHT ARROW in racing modes to change the camera distance.
Press + or - in editing mode to create less or more interpolated curves.
Use your mouse to control the car. Up/Down = Acceleration. Left/Right = Turn.
Applet:
Source Code: p1f.pde
Demo Video: p1f-demo-divx.avi (5.8 MB, DivX Required)
Demo Pictures:
(click to enlarge)
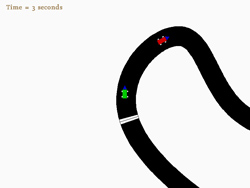
The timer starts when the race begins.
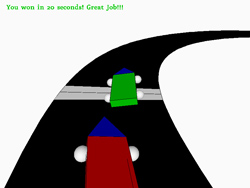
The green human controlled car crosses the finish line first.
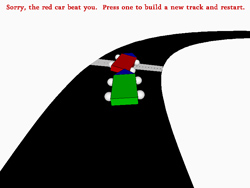
The red computer controlled car crosses the finish line first.